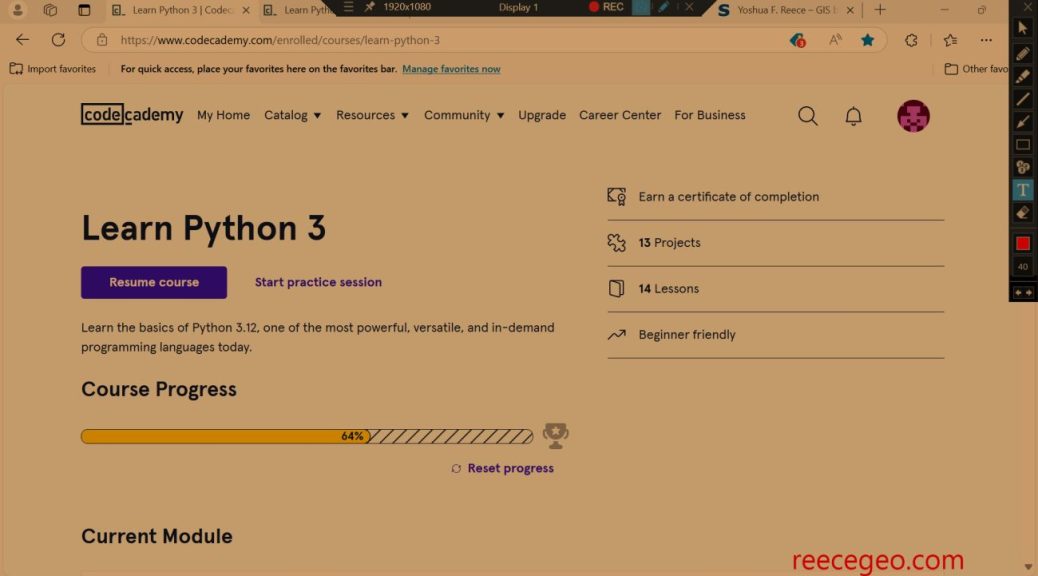
Python Course Project: Strings
For this project, I used string methods to extract information from sales data which was stored as one huge string. I extracted customer names, sales amounts, and product colors. I calculated the total sales and counted the number of each product color sold.
#replace the artifact between each piece of data within a transaction with something without a comma
daily_sales_replaced = daily_sales.replace(';,;', ';')
# split up daily_sales into a list of individual transactions
daily_transactions = daily_sales_replaced.split(',')
#print(daily_transactions)
#split each individual transaction into a list of its data points.
daily_transactions_split = []
for elem in daily_transactions:
daily_transactions_split.append(elem.split(';'))
#print(daily_transactions_split)
#strip off any whitespace from each data item
transactions_clean = []
for elem in daily_transactions_split:
new_list2 = []
for point in elem:
new_list2.append(point.replace("\n", "").strip(" "))
transactions_clean.append(new_list2)
#print(transactions_clean)
#collect the individual data points for each transaction in these 3 lists.
customers = []
sales = []
thread_sold = []
for elem in transactions_clean:
customers.append(elem[0])
sales.append(elem[1])
thread_sold.append(elem[2])
#print(customers)
#print(sales)
#print(thread_sold)
# how much money was made in a day
total_sales = 0
for value in sales:
total_sales += float(value.strip('$'))
#print(total_sales)
#determine how many of each color thread we sold today. Separate any strings for multiple colors separated by an &.
thread_sold_split = []
for item in thread_sold:
if '&' not in item:
thread_sold_split.append(item)
else:
for color in (item.split('&')):
thread_sold_split.append(color)
#print(thread_sold_split)
#function which returns the number of entries in the list that match the argument.
def color_count(color):
return thread_sold_split.count(color)
#print(color_count('white'))
colors = ['red', 'yellow', 'green', 'white', 'black', 'blue', 'purple']
msg = "There were {} {} sold today"
msg2 = "The total value of all sales is {}"
#print statements about the total number of each color sold.
for item in colors:
print(msg.format((color_count(item)), item))