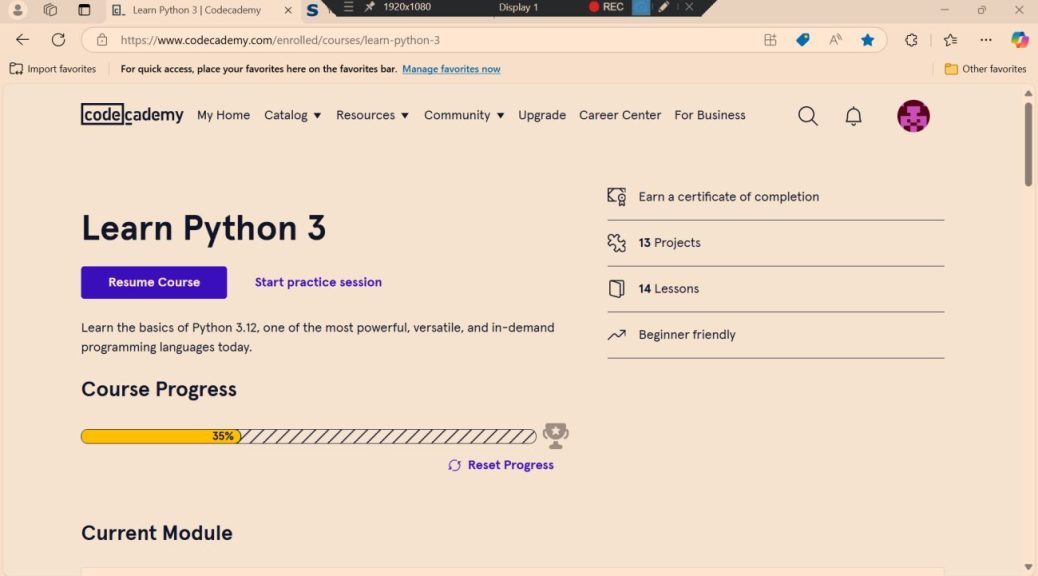
CodeCademy Python Course Update 3
In this guided project, I analyzed data for a hair salon.
hairstyles = ["bouffant", "pixie", "dreadlocks", "crew", "bowl", "bob", "mohawk", "flattop"]
prices = [30, 25, 40, 20, 20, 35, 50, 35]
last_week = [2, 3, 5, 8, 4, 4, 6, 2]
# Calculate total price of all haircuts
total_price = 0
for price in prices:
total_price += price
# Calculate average price of a haircut
average_price = (total_price / len(prices))
print("Average Haircut Price:", average_price)
# Reduce each price by $5
new_prices = [price - 5 for price in prices]
print("New Prices", new_prices)
# Calculate total weekly and daily revenue
total_revenue = 0
for i in range(len(hairstyles)):
total_revenue += (prices[i] * last_week[i])
print("Total Revenue", total_revenue)
print("Average Daily Revenue", (total_revenue / 7))
# Find haircuts under 30
cuts_under_30 = [hairstyles[i] for i in range(len(hairstyles)) if new_prices[i] < 30]
print("Haircuts Under $30", cuts_under_30)