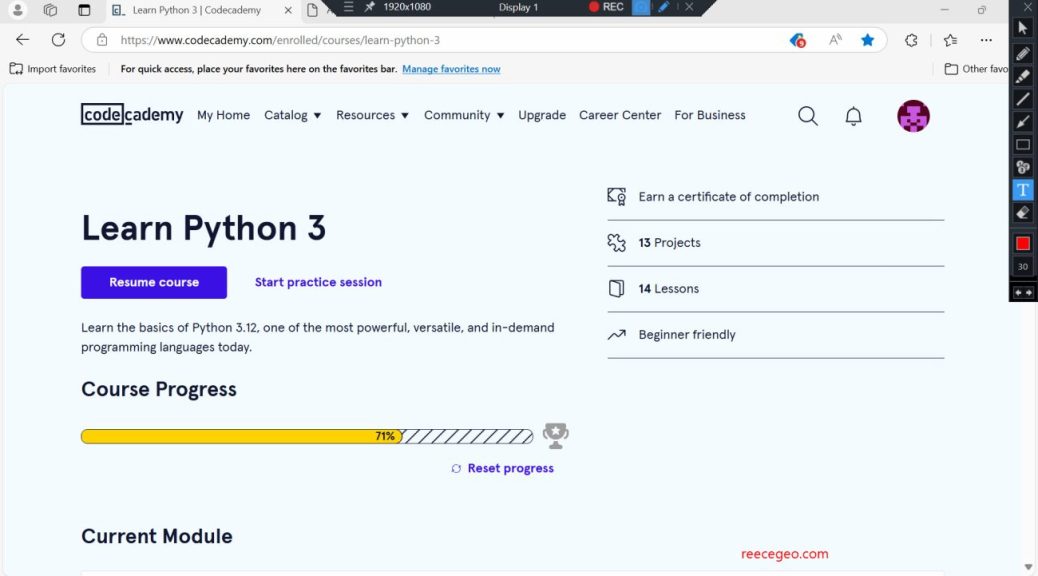
Python course: modules
In this project, I created a Python script which generates a personalized message for a time traveler. The message will read, Today’s date is: 2025-02-02 The current time is: 04:04:19.913236 Pack your bags! You’re traveling to Tokyo in the year 1343. The cost of this trip will be $2387.00.
In this project, I used the decimal, random and datetime modules in Python.
import datetime as dt
from decimal import Decimal
from random import randint
from random import choice
import random
#print the current date and time for reference
current_date = dt.datetime.today().date() # get the current date
current_time = dt.datetime.now().time() # Get the current time
print(f"Today's date is: {current_date}")
print(f"The current time is: {current_time}")
#Randomly determine the destination year and calculate the cost
base_cost = Decimal('3.50') #cost per year to time travel
current_year = dt.datetime.now().year #get current year
target_year = random.randint(1, 2024) #select a random year
year_difference = abs(current_year - target_year) #years traversed
final_cost = base_cost * year_difference
final_cost_round = round(final_cost, 2) #rounded to 2 decimal places
#Randomly determine a destination
destinations = ["Bellingham", "Tokyo", "Albuquerque", "Vancouver", "Daejeon", "Berea", "Da Nang", "Thai Nguyen"]
destination = random.choice(destinations)
#create a message about the time travel experience.
def generate_time_travel_message(year, destination, cost):
return f"Pack your bags! You're traveling to {destination} in the year {year}. The cost of this trip will be ${cost}."
print(generate_time_travel_message(target_year, destination, final_cost_round))