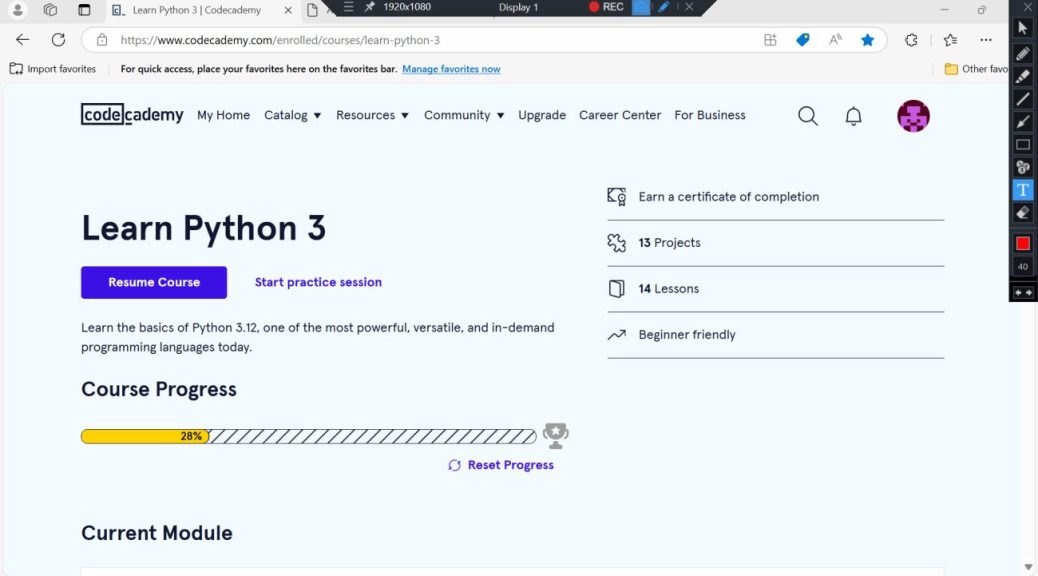
CodeCademy Python Course Update 2
I am learning about lists in Python, and this code I wrote for my latest project in the course I’m taking.
toppings = ["pepperoni", "pineapple", "cheese", "sausage", "olives", "anchovies", "mushrooms"]
prices = [2, 6, 1, 3, 2, 7, 2]
#find out how many $2 slices we have
num_two_dollar_slices = prices.count(2)
#print(num_two_dollar_slices)
#how many kinds of pizza do we have?
num_pizzas = len(toppings)
#print("we sell", num_pizzas, "different kinds of pizza!")
#two dimensional list of pizzas and prices
pizza_and_prices = [[2, "pepperoni"], [6, "pineapple"], [1, "cheese"], [3, "sausage"], [2, "olives"], [7, "anchovies"], [2, "mushrooms"]]
#print(pizza_and_prices)
#sort the pizzas in order of ascending price
pizza_and_prices = sorted(pizza_and_prices)
#print(pizza_and_prices)
#store the cheapest pizza
cheapest_pizza = pizza_and_prices[0]
#print(cheapest_pizza)
#store the most expensive pizza
priciest_pizza = pizza_and_prices[-1]
#print(priciest_pizza)
#remove a slice from list
pizza_and_prices.pop(-1)
#print(pizza_and_prices)
#add a slice to the list
pizza_and_prices.insert(4, [2.5, "peppers"])
#print(pizza_and_prices)
#slice the three lowest-price pizzas
three_cheapest = pizza_and_prices[:3]
print(three_cheapest)