At the finish line
The project is almost complete! I used chatGPT to help me create a simple GUI with TKinter, and then used PyInstaller to bundle everything up into an executable (.exe) file. This self-contained executable can be stored on a flash drive and run on any computer without installing. I had read that portable apps can be slower-performing due to the speed of usb information transfer, however i don’t notice a difference after trying it.
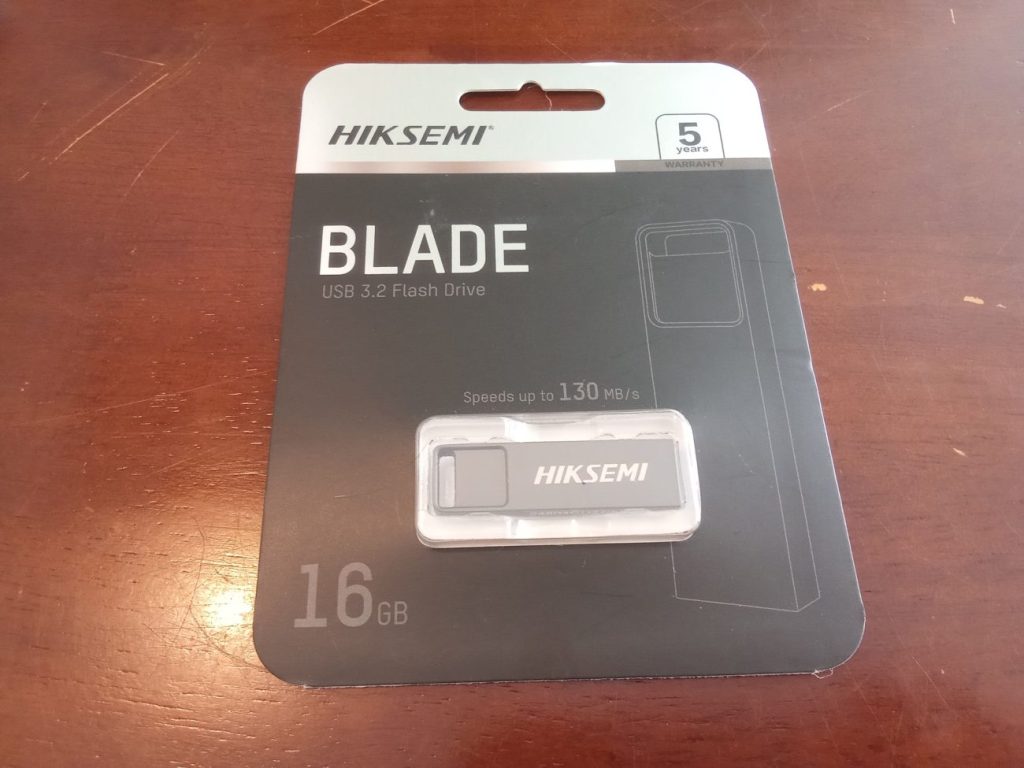
The UX is just how I wanted. The user can just double click the .exe file to launch the GUI, select a video file and click “transcribe”, the .srt file automatically opens in notepad, the user proofreads and translates, then saves and closes, and clicks “add to video”. The subtitled mp4 video appears in the output folder.
I had some trouble with Windows Defender flagging the executable as malware. The name of the supposed malware is Trojan:Win32/Wacatac.B!ml. I did a little bit of reading, and it seems that this is commonly a false positive when developing apps in python. Still, I want to be able to distribute this file to my coworkers and for them to not worry.
I digitally signed the app by creating a self-signed certificate. I’ll include it with the .exe file when sharing, so users can double click the .cer to open a wizard and add it to their trusted store (thereby telling their system to trust the app and not give malware warnings). I also created a text document explaining and proving instructions about this.
I also submitted the file to Microsoft Security Intelligence for Malware Analysis. This will give me more assurance that the file isn’t malware. I’m still waiting for the results, which can take a few days.